TABLE OF CONTENTS
Redux is a state management library for JavaScript apps. In this article, we will walk over React-Redux and how to use it. We will use it to simplify the complex state management of membership and authentication flows.
Redux is a state management library for JavaScript apps. It helps us put all of the application states in a global variable called the store. It is the most popular package for managing state in the React ecosystem. For this reason, there is a dedicated package called React-Redux for React.js apps.
Redux makes it easier to manage the state of large web apps. This could be a membership site, social network, or an e-commerce site. Normally such sites have a lot of components that need to share the same state.
In this article, we will walk over React-Redux and how to use it. We will use it to simplify the complex state management of membership and authentication flows.
What is React-Redux?
At a high level, redux is a global state management library for JavaScript apps. React Redux will offer us a central store to hold all the React application states. It has an API so any component at any level of the app can access and update the state at any time.
In react apps, every component can have its own local state. React will re-render the view every time the state changes.
React Hooks Example
A state is like a simple property like a count value, or a color. Defining this property in the state makes it a special variable. React will keep track of this state.
Our example application has a color state set to black. Every local state can start with a default value, and a method to update it.
- Using the useHook() method, we are setting a color variable.
- With the updater method, setColor we can change the color.
Now we are setting the color in the JSX with a style tag.
Next, we will hook this setColor() method with a button. This is called an event listener. With it, we can change the color with a click of a button.
When we click the button the color will change. Internally, we are just changing the state from yellow to blue.
First Hook Example:
Since React is tracking the change, it will re-render automatically for us. React is set up in a way that the component view will change whenever it detects a state change.
React Redux does this in a different way. Instead of storing the state locally, it puts everything globally in one place. So all the state stays outside of the presentation layer.
In a simple hello world app like this, we would't have to use Redux. But when our app grows bigger, we'll find redux very useful.
When to Use Redux in React?
You will need React Redux when you build a large application with lots of components. It is needed when each of these components want to access a shared state.
Sibling components cannot access the state of each other since React has one-way data binding. One-way-data binding means React can only pass the data downwards. A way to go around this is by lifting the state. By doing so, a parent component holds the state for children to use.
This is completely fine for simple hello world apps. But if you are making a real application, then passing state and methods can become a daunting task. React Redux makes it easier to access the global state from any component.
In short, when you are building a big application with lots of states, you will need Redux. Redux is a global state management library allowing components to share states with each other.
Real World Use Case of Redux
Let's imagine a membership site. The site is saving the logged-in member detail in the root component of the app. Now, it's common for logged-in members to update their account page, make comments, and post on the site.
Our members can have information like their name, username, and avatar. Inside the app, it is usually stored in the root component. But other parts of the app like the account page, comments section, and article page may also want to display them.
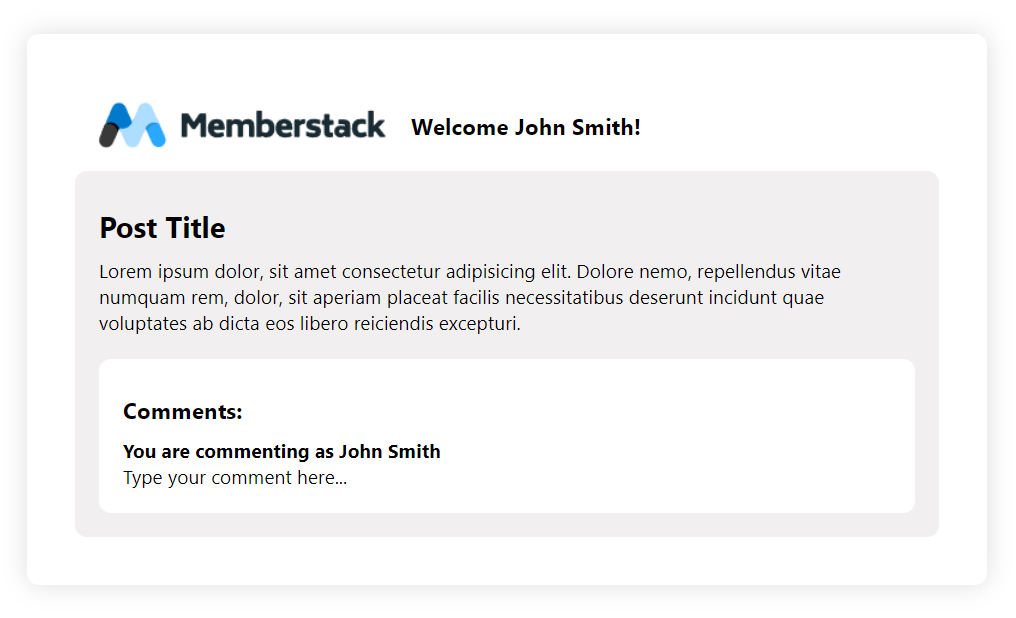
Under the hood, the fictional React application will have these components:
- Root component
- Members area
- Account page
- Article page
- Comment component, etc.
Each component will need to have access to the piece of info they want to display. If the user info is stored in the Root, then we have to pass it to every component one by one. You can start to see why managing a local state sucks!
React Hooks Membership Site Example
Let's go back to our hello world example. We now have a header component for displaying the header. We want to put the button we had in the body section in the header. So we can control the color right from the header.
Since React has one-way data binding, it's not possible for the Header to access the state inside the Body. We have to put this state in a parent component.
In this rewrite, we will move the color state into the App component. Now it becomes the local state of the App component. We will pass the color state from App to Body. This will allow the Body to access the color.
We will also pass the setColor method from App to Header as a prop. Because we want the header to have the switch that changes color. Now we can safely move the button from the Body to the Header.
When we run this app, we are now controlling the color of the body from the header section.
Lifting the state:
In reality, the Body component does not have its own color state, it is using its parent state. In the same way, there is no setColor method in the Header, it's coming from its parents.
In the real world, our app will have even more deeply nested components. How would we pass them down below?
- The Body may have an inner component for Post.
- The Post may have another one for Comment.
When we want to send the local state down to the deeply nested children component, it's called prop drilling.
We would do something like this:
Prop Drilling:
Each of the child components passes prop to the next child components.
Can you see how difficult it is to manage working with a local state? You should be able to finally see the benefits of having a global state using React-Redux.
Now let's move on to a more hands-on guide for using Redux in your React apps.
How to install React Redux?
If you are starting a new React project with Create-React-App, then add Redux with the template. If you are adding redux to an existing project, you have to install it manually.
Redux Toolkit is the new and recommended pattern for working with Redux in React apps. Here's a breakdown of the two ways you can install Redux in your React application:
How to Install Redux with Create React App:
Here, we are creating a brand new project with Create React App. We will install Redux Toolkit using the template:
This will generate a fresh copy of React along with the required files. It will use redux for state management instead of hooks.

Redux will make the app with a counter-app example. When we open the app, we can see all the features of Redux Toolkit.
How to Install Redux to an Existing React App:
To install Redux to an existing React site, install these two packages:
- @reduxjs/toolkit
- react-redux
You can use either npm or yarn to install them.
We are going to install them using the yarn package manager.
Redux Toolkit is the new recommended pattern of working with Redux. React-redux is what binds Redux with ReactJS apps.
After installing these packages, we will also create a few files. We will go through them in the following tutorial section.
Configuring Redux Toolkit with an Existing React Application
We will first install these dependencies with npm or yarn:
or
1. Create a Redux Slice
A slice file is a slice of the global state we will create. This will help us manage the states more easily.
Redux Toolkit recommends we create the slice files under src/features/ folder. We will create a color slice to change the color of the body. Let's create the slice file called colorSlice.js under src/features/color/colorSlice.js
Step 1. Import the createSlice method. So we can create slices.
Step 2. Make the initial state with default color.
Step 3. Create the colorSlice using the createSlice method.
This method takes the name of the slice, the initial state, and the reducers.
Reducers are the methods we will call from our component to make changes to the state.
For our first method, we will create a changeToBlue method to change color.
This method takes a state parameter and then allows us to update it.
Step 4. Export the actions for our application:
Step 5. Export the reducer as the default export:
The final code file at this point looks like this:
2. Create a Store and add the Slice
After creating the slice, we will now create a store and add our color slice reducer to it. A store is like a global variable that holds all the application states.
Step 1. Create store.js file under src/app/store.js
Step 2. Import configureStore method from redux toolkit:
Step 3. Import colorReducer from the color slice file:
Step 4. Create the store using the configureStore method:
Step 5. Add the color reducer slice as a property in store:
The final store.js file with the reducer will look like this:
3. Connect the store to Your App
After our store is ready, we will now have to connect the store. This will inform React about Redux. It will make all the states available to every component of our app.
Step 1. Open index.js from React root directory:
Step 2. Import the store file we created:
Step 3. Import the Provider from react-redux. It will connect redux with React:
Step 4. Wrap <App/> component with <Provider> component. Provide store prop and link to the store:
The final code will look like this:
4. Access Redux inside Component
Now that we have completed the setup, it's time to use Redux. We will open a component and these steps.
Step 1. Import useSelector and useDispatch from react-redux:
useSelector will help us access the color state. useDispatch will help us call redux to change the color action.
Step 2. Import changeToBlue action from the colorSlice file:
Step 3. Set the color value inside the component:
Step 4. Create a dispatch method by using useDispatch:
Step 5. Add color to our app:
Step 6. Hook up the dispatch method with the changeToBlue action with a button:
Full code of this component file:
After hooking up our app with Redux, we can easily change the color of the component by clicking a button just like in the previous example.
With a simple app like this, it's too much configuration. However, when you build a large website, you will gain productivity in the long run. Let's look at an example.
Redux Toolkit Membership Site Example
On our membership site, we will get our logged-in user info and save them in the state. We will create a slice to store this logged-in member information:
We can set our initial state to empty when no one is logged in:
When someone logs in we can save their info like this:
Inside our reducers, we can create an action setUser to handle setting up user info:
We can then call this action from the login section of our application.
The login section will make a new user object and set it to store using dispatch:
After the user information is set in the global store, any component can use it with useSelector.
If we have a comment component, we can get the user info like this:
React Hooks vs Redux Toolkit, Which one to use?
React Hooks should be your first approach to state management. It is built-in to React so no need to download anything. Use hooks for these situations:
- Your site has a handful of components.
- Not many deeply nested components.
- Your app does not need to access the shared state.
On the other hand, Redux is good but takes a lot of setup to start. Use Redux in these situations:
- You have a dynamic site.
- Lots of components depend on each other.
- Many deeply nested components want to access the root state.
We would suggest you start with hooks. After that, when you see your application is getting complicated, introduce redux.
Conclusion
Redux is a state management tool for React. Redux is needed when you are building a large web application like a membership site. To summarize what we learned so far:
- React uses hooks to store state locally.
- Redux uses a global store for state.
- With Redux you can access state from any component.
- Create-React-App has a redux template to setup automatically.
- Redux Toolkit is the new pattern for using Redux in React.
- We use redux for long-term productivity gains.