Commenting for Webflow is LIVE on Product Hunt! Get 50% off for 12 months →
Competition: Build a SaaS app between May 29 - June 14 and get a chance to win cash prizes, free plans & more!
#111 - Auto Rotating Tabs v0.1
The easiest way to make your tabs auto-rotate on a timer.
View demo
<!-- 💙 MEMBERSCRIPT #111 v0.1 💙 - AUTO-ROTATING TABS -->
<script>
// Function to rotate tabs
function initializeTabRotator() {
// Find all tab containers with the ms-code-rotate-tabs attribute
const tabContainers = document.querySelectorAll('[ms-code-rotate-tabs]');
tabContainers.forEach(container => {
const interval = parseInt(container.getAttribute('ms-code-rotate-tabs'), 10);
const tabLinks = container.querySelectorAll('.w-tab-link');
const tabContent = container.closest('.w-tabs').querySelector('.w-tab-content');
const tabPanes = tabContent.querySelectorAll('.w-tab-pane');
let currentIndex = Array.from(tabLinks).findIndex(link => link.classList.contains('w--current'));
let rotationTimer;
// ANIMATION CONFIGURATION
// Modify these values to adjust the animation behavior
const FADE_OUT_DURATION = 300; // Duration for fading out the current tab (in milliseconds)
const FADE_IN_DURATION = 100; // Duration for fading in the new tab (in milliseconds)
const EASING_FUNCTION = 'ease'; // Choose from: 'linear', 'ease', 'ease-in', 'ease-out', 'ease-in-out'
// or use a cubic-bezier function like 'cubic-bezier(0.1, 0.7, 1.0, 0.1)'
// Additional easing options (uncomment to use):
// const EASING_FUNCTION = 'ease-in-quad';
// const EASING_FUNCTION = 'ease-out-quad';
// const EASING_FUNCTION = 'ease-in-out-quad';
// const EASING_FUNCTION = 'ease-in-cubic';
// const EASING_FUNCTION = 'ease-out-cubic';
// const EASING_FUNCTION = 'ease-in-out-cubic';
// const EASING_FUNCTION = 'ease-in-quart';
// const EASING_FUNCTION = 'ease-out-quart';
// const EASING_FUNCTION = 'ease-in-out-quart';
// const EASING_FUNCTION = 'ease-in-quint';
// const EASING_FUNCTION = 'ease-out-quint';
// const EASING_FUNCTION = 'ease-in-out-quint';
// const EASING_FUNCTION = 'ease-in-sine';
// const EASING_FUNCTION = 'ease-out-sine';
// const EASING_FUNCTION = 'ease-in-out-sine';
// const EASING_FUNCTION = 'ease-in-expo';
// const EASING_FUNCTION = 'ease-out-expo';
// const EASING_FUNCTION = 'ease-in-out-expo';
// const EASING_FUNCTION = 'ease-in-circ';
// const EASING_FUNCTION = 'ease-out-circ';
// const EASING_FUNCTION = 'ease-in-out-circ';
// const EASING_FUNCTION = 'ease-in-back';
// const EASING_FUNCTION = 'ease-out-back';
// const EASING_FUNCTION = 'ease-in-out-back';
// END OF ANIMATION CONFIGURATION
function switchToTab(index) {
// Fade out current tab
tabPanes[currentIndex].style.transition = `opacity ${FADE_OUT_DURATION}ms ${EASING_FUNCTION}`;
tabPanes[currentIndex].style.opacity = '0';
setTimeout(() => {
// Remove active classes and update ARIA attributes for current tab and pane
tabLinks[currentIndex].classList.remove('w--current');
tabLinks[currentIndex].setAttribute('aria-selected', 'false');
tabLinks[currentIndex].setAttribute('tabindex', '-1');
tabPanes[currentIndex].classList.remove('w--tab-active');
// Update current index
currentIndex = index;
// Add active classes and update ARIA attributes for new current tab and pane
tabLinks[currentIndex].classList.add('w--current');
tabLinks[currentIndex].setAttribute('aria-selected', 'true');
tabLinks[currentIndex].setAttribute('tabindex', '0');
tabPanes[currentIndex].classList.add('w--tab-active');
// Fade in new tab
tabPanes[currentIndex].style.transition = `opacity ${FADE_IN_DURATION}ms ${EASING_FUNCTION}`;
tabPanes[currentIndex].style.opacity = '1';
// Update the data-current attribute on the parent w-tabs element
const wTabsElement = container.closest('.w-tabs');
if (wTabsElement) {
wTabsElement.setAttribute('data-current', tabLinks[currentIndex].getAttribute('data-w-tab'));
}
}, FADE_OUT_DURATION);
}
function rotateToNextTab() {
const nextIndex = (currentIndex + 1) % tabLinks.length;
switchToTab(nextIndex);
}
function startRotation() {
clearInterval(rotationTimer);
rotationTimer = setInterval(rotateToNextTab, interval);
}
// Add click event listeners to tab links
tabLinks.forEach((link, index) => {
link.addEventListener('click', (e) => {
e.preventDefault();
switchToTab(index);
startRotation(); // Restart rotation from this tab
});
});
// Start the initial rotation
startRotation();
});
}
// Run the function when the DOM is fully loaded
document.addEventListener('DOMContentLoaded', initializeTabRotator);
</script>
Creating the Make.com Scenario
1. Download the JSON blueprint below to get stated.
2. Navigate to Make.com and Create a New Scenario...

3. Click the small box with 3 dots and then Import Blueprint...

4. Upload your file and voila! You're ready to link your own accounts.
Need help with this MemberScript?
All Memberstack customers can ask for assistance in the 2.0 Slack. Please note that these are not official features and support cannot be guaranteed.
Join the 2.0 SlackVersion notes
Attributes
Description
Attribute
No items found.
Guides / Tutorials
Tutorial
What is Memberstack?
Auth & payments for Webflow sites
Add logins, subscriptions, gated content, and more to your Webflow site - easy, and fully customizable.
Learn more
.webp)
"We've been using Memberstack for a long time, and it has helped us achieve things we would have never thought possible using Webflow. It's allowed us to build platforms with great depth and functionality and the team behind it has always been super helpful and receptive to feedback"

Jamie Debnam
39 Digital
"Been building a membership site with Memberstack and Jetboost for a client. Feels like magic building with these tools. As someone who’s worked in an agency where some of these apps were coded from scratch, I finally get the hype now. This is a lot faster and a lot cheaper."

Félix Meens
Webflix Studio
"One of the best products to start a membership site - I like the ease of use of Memberstack. I was able to my membership site up and running within a day. Doesn't get easier than that. Also provides the functionality I need to make the user experience more custom."

Eric McQuesten
Health Tech Nerds
Off World Depot
"My business wouldn't be what it is without Memberstack. If you think $30/month is expensive, try hiring a developer to integrate custom recommendations into your site for that price. Incredibly flexible set of tools for those willing to put in some minimal efforts to watch their well put together documentation."

Riley Brown
Off World Depot

"The Slack community is one of the most active I've seen and fellow customers are willing to jump in to answer questions and offer solutions. I've done in-depth evaluations of alternative tools and we always come back to Memberstack - save yourself the time and give it a shot."
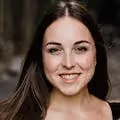
Abbey Burtis
Health Tech Nerds
Slack
Need help with this MemberScript? Join our Slack community!
Join the Memberstack community Slack and ask away! Expect a prompt reply from a team member, a Memberstack expert, or a fellow community member.
Join our SlackTry Memberstack for free
100% free, unlimited trial — upgrade only when you're ready to launch. No credit card required.
Product
Full Feature ListUser AccountsGated ContentSecure PaymentsAPI & IntegrationsMemberstack & WebflowMemberstack & WordPressCreate a new account2.0 Log in1.0 Log inPricingLanguage
Privacy PolicyTerms of ServiceCookie PolicySecurity Policy
© Memberstack Inc. 2018 – 2025. All rights reserved.